- Home
- Web Development
- Beyond the Basics: Mastering S ...
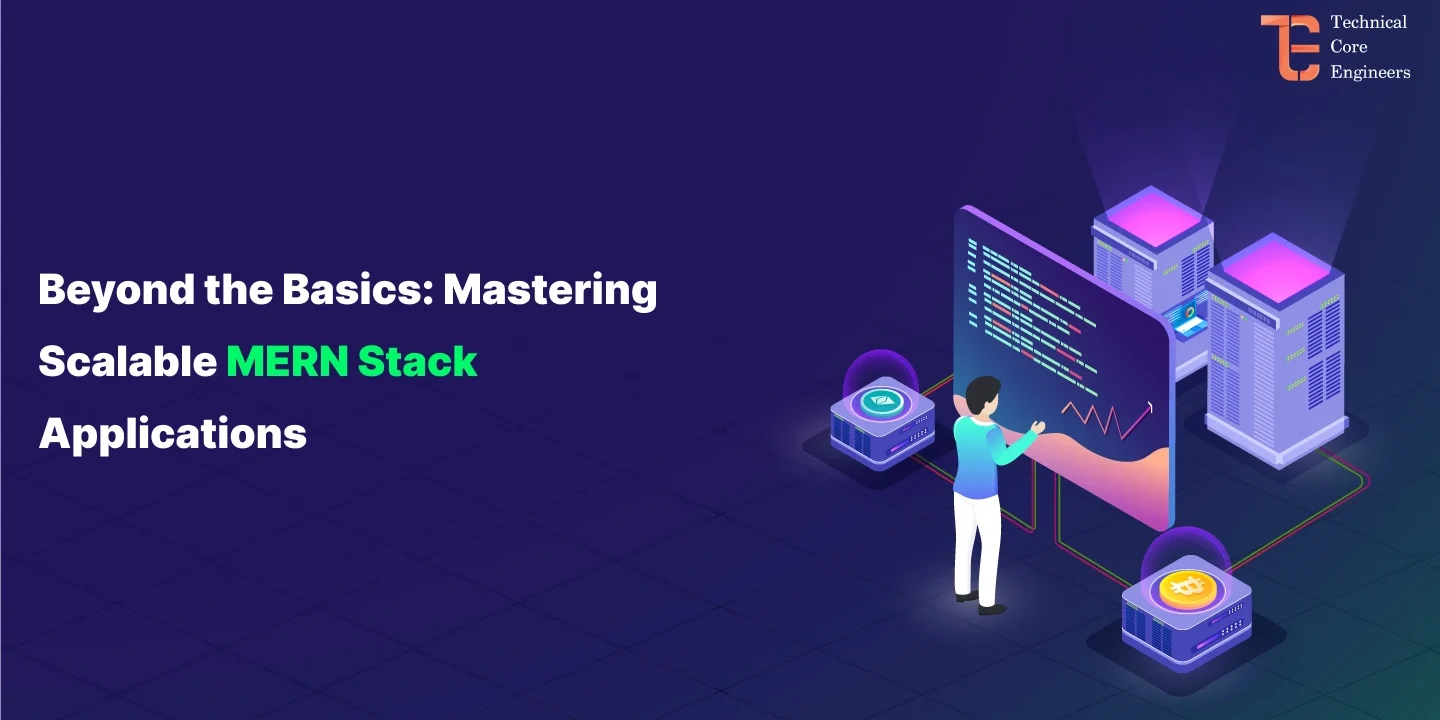
Introduction
In today’s fast-paced digital landscape, building a web application that simply works isn’t enough. You need scalability, efficiency, and high performance to handle growing user demands. The MERN (MongoDB, Express.js, React, and Node.js) stack is a powerful choice, but mastering it for scalability requires a strategic approach.
In this guide, we’ll explore key techniques to scale your MERN stack application while keeping it optimized and high-performing. Whether you’re a startup looking to future-proof your app or an enterprise handling millions of users, these best practices will set you on the right path.
Understanding MERN Stack Scalability
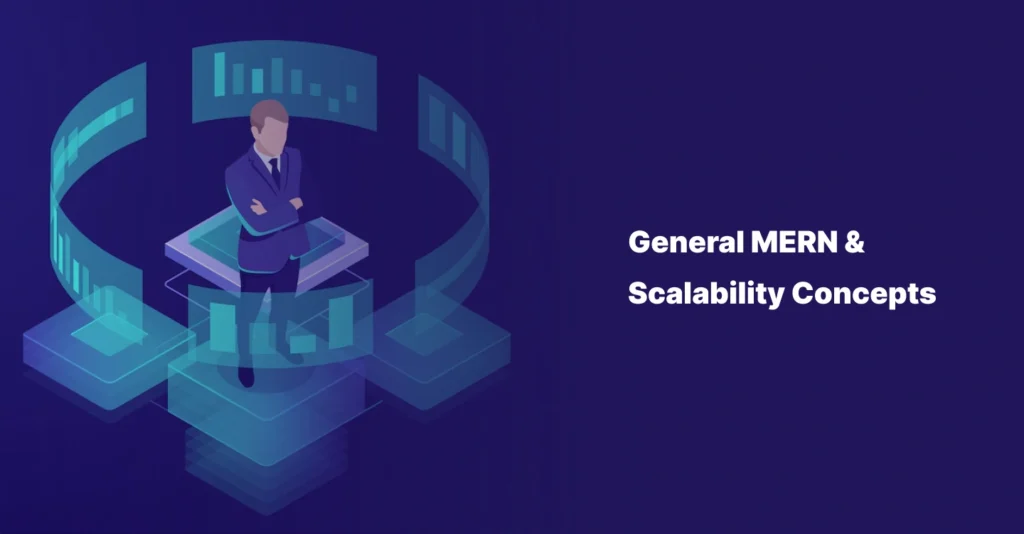
Why Scalability Matters
Scalability ensures your application can handle increasing traffic, data, and workload efficiently without compromising performance. A well-scaled MERN application can:
- Manage high user requests seamlessly
- Improve load times and responsiveness
- Reduce server costs with optimized resource usage
- Maintain high availability and reliability
Let’s break down the best practices for each layer of the MERN stack.
Database & Backend Optimization
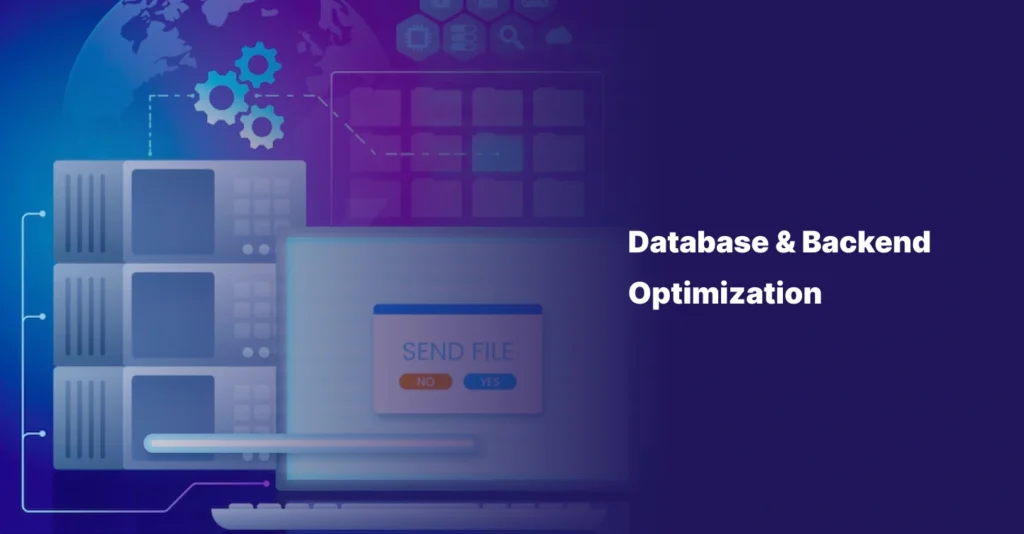
1. MongoDB Sharding
As your database grows, queries can slow down. Sharding helps distribute data across multiple servers, ensuring better read and write performance.
📌 Example: Imagine a social media platform storing millions of user posts. Instead of keeping everything in one database, sharding can distribute posts across multiple servers based on user IDs.
How to Implement:
- Use hashed sharding for even distribution.
- Deploy a config server to manage shard metadata.
- Balance load with query routing using
mongos
.
2. MongoDB Replication
Replication ensures high availability by keeping multiple copies of your database.
Best Practices:
- Use a Replica Set to maintain backups.
- Automatically failover to secondary nodes when the primary node is down.
- Keep at least three replica nodes for data redundancy.
3. Indexing for Faster Queries
Indexes dramatically improve database performance by reducing query time.
✅ Example: Instead of scanning an entire user collection, an index on email
allows instant lookups.
Command:
db.users.createIndex({ email: 1 });
4. Using Caching (Redis)
Redis stores frequently accessed data in-memory, reducing database load.
📌 Example: Cache user authentication sessions instead of querying MongoDB every time.
Implementation:
const redisClient = require('redis').createClient();
redisClient.set('user:123', JSON.stringify(userData));
Frontend Performance Optimization
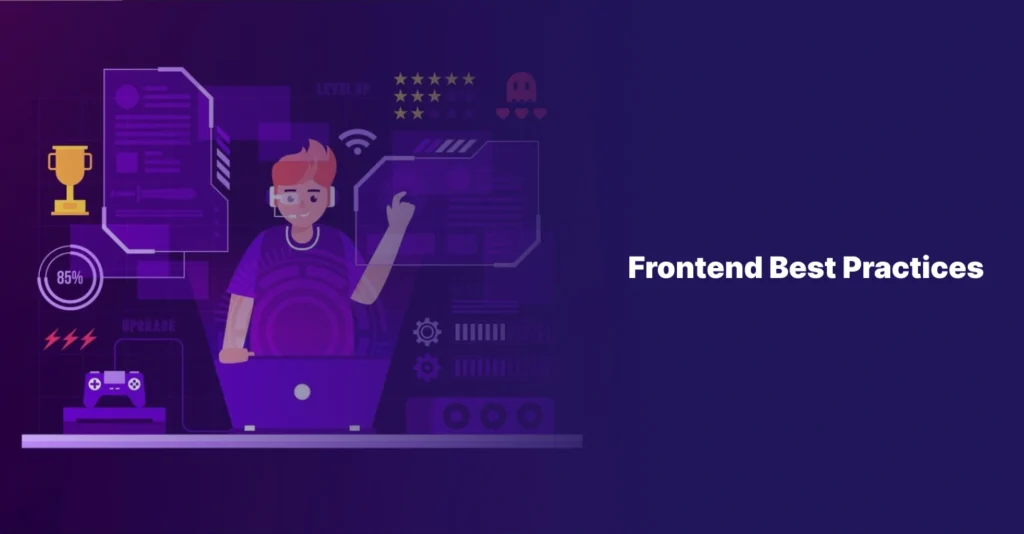
5. Code Splitting & Lazy Loading
React applications can become sluggish with large JavaScript bundles. Code splitting ensures users only load what they need.
📌 Example: Split dashboard and user profile components to load separately.
Implementation:
const Dashboard = React.lazy(() => import('./Dashboard'));
6. Optimized State Management
Use Redux Toolkit or React Query for efficient state handling.
Best Practices:
- Minimize global state where possible.
- Use memoization to avoid unnecessary renders.
- Normalize data to reduce redundant API calls.
7. Server-Side Rendering (SSR) for Faster Loads
Next.js enables SSR, improving SEO and load times.
📌 Example: Pre-render product pages instead of fetching them client-side.
export async function getServerSideProps() {
const data = await fetchAPI();
return { props: { data } };
}
Infrastructure & DevOps for Scaling
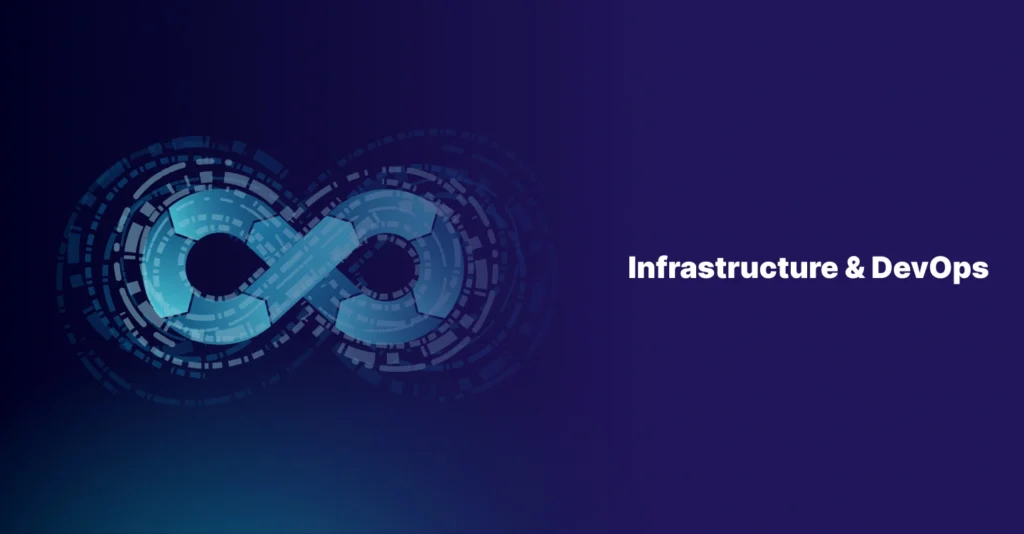
8. Dockerized MERN Applications
Containerizing your MERN app ensures consistency across environments.
Dockerfile Example:
FROM node:18
WORKDIR /app
COPY . .
RUN npm install
CMD ["node", "server.js"]
9. Kubernetes for Auto Scaling
Kubernetes helps manage deployments and auto-scale based on demand.
Best Practices:
- Use Horizontal Pod Autoscaling (HPA).
- Deploy load balancers to distribute traffic.
- Monitor performance with Prometheus & Grafana.
10. CI/CD Pipelines for Faster Deployment
CI/CD automates testing and deployment, ensuring reliability.
📌 Example: Automate deployment on AWS/GCP after pushing code to GitHub.
Tools to Use:
- GitHub Actions or Jenkins for CI/CD
- Docker Hub for container registry
- Kubernetes clusters for managed deployment
Conclusion
Scaling a MERN stack application isn’t just about handling more users—it’s about creating a robust, high-performing, and cost-efficient system. By implementing database optimizations, frontend best practices, and DevOps strategies, you ensure your application remains smooth and scalable as it grows.
Start implementing these techniques today and take your MERN stack applications beyond the basics! 🚀
What’s Next? Looking to build a scalable MERN stack project? Need expert assistance? Get in touch with us today!